How Programs Run¶
Read time: 38 minutes (9734 words)
Since you should have already taken an introductory course in computer programming, you should know some of the basics of the modern computer is organized. Basically, we will be managing a processor, and code and data locate din the system’s memory. We will not worry much about how the processor actually works, that is the subject of a course like COSC2325: Computer Architecture and Machine Language (which has been my favorite course for over 15 years now!)
We do need to figure out how we will create programs using C++?
Classifying Languages¶
There are two major classes of programs around today: Those that are “interpreted” and those that are “compiled”. Let’s take a quick look at each.
Interpreted Languages¶
Some languages are processed by a special program called an “interpreter”. That tool reads your code, figures out what is supposed to happen, then makes that happen. This is done line by line as your code file is read and processed. This approach to programming is slow, since the program code may be read over and over if needed, but development is quick, since the machine cna process your code immediately (well, as soon as you save the file on your system somewhere.)
May old(er) programmers learned their craft using an ancient language called Basic which worked exactly just this way. (Bill Gates wrote a Basic language tool before he founded Microsoft!)
Compiled Programs¶
If you want your code to run as fast as possible, nothing beats using a “compiled” language like C++. In these languages, a special tool called a “compiler” reads your program and translates it into the native language of the machine you plan to run the program on. Note that the target machine may not be the machine you use to process your code. I regularly write C++ code on my Mac, and run that code on tiny microcontroller processors designed to be buried in some consumer gadget!
Compilers can spend some time working on your program to make is as fast as possible. This is called “optimizing” your code. In the end, a good compiler will translate your code into a nice form, ready for important stuff like blasting space aliens!
Hybrid Languages¶
Actually, Python (and Java) falls in the middle, between these two major classes of languages. Both of these languages translate your program into something called “Byte-Code” which is a simple set of codes for an ideal computer. Then a special program called a “byte-code interpreter” runs that byte-code to make your program run. The advantage of this is better performance than a pure interpreter, and a simple way to build a tool that can run these languages on any system. All you need to do is write a “byte-code interpreter” for the new machine, and you are ready to run!
Note
Just so you know, The Python “byte-code interpreter” is written in C++.
Compiling C++ Programs¶
Most of you took COSC1336 before this class, and learned the basics of programming using Python. While Python is a great language for a number of applications, it does have one big failing: it can be slow! In today’s fast paced world, we need more speed! We want answers NOW, not later, so we must move to a different language, and a different way of processing that language to get the speed we want.
C++, like many other high-level languages, is a “compiled language”. In these languages, a special program called a “compiler” reads your program source code, checks that it obeys the rules for writing a program in that language, then translates your code into a set of instructions for one particular computer. Those instructions, called “machine instructions” are built into the processor. The compiler works hard to make sure the resulting set of instructions will run as fast as is possible on that particular computer.
This results in very fast programs, but there is one problem. If you want to run the same program on a different computer (with a different processor or operating system), you need to find a compiler for that particular system and process your program again.
Python can be run on all kinds of machines easily, but you still need to install Python on each machine you want to use.
The Actual Programming process¶
In this class, we will follow a series of steps to build and run your programs. You will use a number of tools and produce a number of “artifacts” that help the tools build a runnable program. Here is the basic process:
Write your Code¶
You start off by using an editing tool, hopefully one that can help you get this done, and create a “source file”. That file may contain only one part of a complete program. You may write several different files which together fully define everything needed for your program. The end result of this step is one or more program source code files, each with a name that is supposed to help you remember what part of your program is where. Most such files have an “extension” indicating what language they are written in. For C++ programs, the common extension is “.cpp”.
Note
You will build files for C++ that end in “.h”, which means these files are “header” files. More on that later.
Compile Your Code¶
Next, you will fire up a C++ “compiler” which does two things:
Checks the “syntax” of your code to make sure you wrote legal C++ code.
Translates that C++ code into a form your computer can actually run
Our compiler translates your code into “assembly language” for the processor in your computer. A second tool, called an “assembler” translates that code into something called an “Object file”, which is almost ready to run on your system
Note
Some compilers, go straight to object file form, and do not use an assembler to help out.
If you have any errors in your syntax, the object files are not produced, and you get to fix syntax errors! Not fun, but necessary when you cannot type very well!
Link Your Object Files¶
Each source code file will resulting an associated object file. We need to tie all these object files together to build a complete program.
Oh yeah, we need one more thing!
C++ Runtime Support¶
Most of your programs will use functions provided with the language. We need for the code for those functions to be included as well as our own object files. In the compiled language world, you do not need the source code for those functions, just the object files resulting from compiling those modules. All of these object files are included with the compiler in the form of a “runtime system”.
“Linking” is the term we use for the action of combining all the parts needed to build a complete program into a single “executable” package.
The linker reads the object files that make up your program. If any object file refers to other functions or variables in other object files, it notes the fact that something is missing in this file, and will later fill in the missing pieces as the linking process proceeds. As other object files are processed, any new missing parts are noted, and any holes that currently exist are filled in where possible This process continues until all object files you wrote have been processed.
Finally, the C++ runtime library is searched for any remaining missing parts. If all missing points are located, the linker builds a final “executable file” which contains everything needed to actually run the program. It is not “executable” directly though, as we will see in a moment.
If the linker finds something is missing, you will get a “link error” saying something like “unresolved external references”. This message tells you some parts are missing, and the program cannot run. No “executable file” will be produced, and you get to figure out what is missing!
Loading Your Code¶
When you have an “executable file” for your program, you can run it as usual. You might “double click” on an icon, or the executable file name to start this last process. You might also type in a command to the operating system asking it to launch your program for you.
No matter how you start the program up, what actually happens is that an operating system component called a “loader program” reds your executable file, and install all the program code and data into memory. The final result is that the processor can finally run your code. That does not actually happen right away in most modern computers. Instead, your program is added to a list of other programs in memory at the same time, and the processor sweeps over all of those programs giving a little bit of its time to each one in turn. The result of this trickery is that you seem to see all of them running at the same time, when actually ony one is running, and only for a short time. The processor makes sure each program is unaware that it is being started and stopped at a furious rate, letting many things seem to happen at the same time!
Magic!
Nope, just fantastically fast processors doing their thing!
Putting it all together¶
Here is a diagram showing how all this ties together:
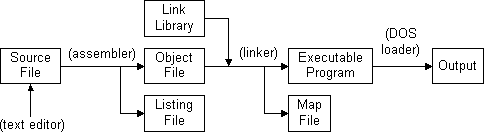
(Substitute compiler where is says assembler.)
Installing Required Tools¶
In the next few lectures (while we will go over briefly in class), you will install the tools you will need to build programs for this class. For now, do not worry much about how to use each one. Just make sure you get these tools set up on the development machine you plan to use for this class. I will have all of the required installation files loaded on the “I” drive on lab machines as soon as I can get to them after the course starts.
Note
You will find that working on programming classes is much easier if you set up a laptop with the tools needed for your class. That way, you can work when and where you wish. If you do not own a laptop, you can use the lab machines, which have all the required tools installed. If you must use a desktop at home, and have problems, you probably should come to my office hours and go over the process if you get stuck.
Here are the tools we will be using this term: